Background
Read background information on the design of the timeclock system!
Single vs. Multiple timesheets
Since each student’s timesheet is formatted the same way, whether it is generated as a single timesheet or as part of multiple timesheets, I developed a class to create the timesheets.
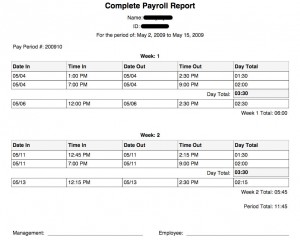
In the class is a function called outputPDF which accepts one argument: an array of the timesheet values. Inside the function we begin creating the page.
function outputFPdf($app_data) { $app_data; ob_start(); $output = null; $output .= $this->fpdf->SetFont('Arial','B',15); $output .= $this->fpdf->Cell(80); $output .= $this->fpdf->Cell(30,10,'Complete Payroll Report',0,0,'C'); $output .= $this->fpdf->Ln(8); $output .= $this->fpdf->SetFont('Arial','',10); $output .= $this->fpdf->Cell('',10,"Name: ".$app_data['name'],0,0,'C'); $output .= $this->fpdf->Ln(6); <---- edited for brevity ----> ob_clean(); return $output; }
Now that we’ve got the timesheet ready we’ll create a function that determines the timesheet type.
There are a few things we want to accomplish with this function.
- Include and instantiate the fpdf library
- Call the FPDF page numbering function and create the first page
- Set the page title
- Check to see if the type is single and if so, generate the timesheet
- If the type isn’t single check to see if it is multiple and if so, generate the timesheets
So all together it looks like this:
function createPdf($app_data, $type, $begin, $end, $section=null) { // include and instantiate the fpdf library include 'includes/fpdf.php'; $this->fpdf = new fpdfHelper(); // Call the FPDF page numbering function and create the first page: $this->fpdf->AliasNbPages(); $this->fpdf->AddPage(); // Set the page title: $this->fpdf->setTitle('Complete Payroll Report'); // check to see if the type is single and if so, generate the timesheet if($type == 'single') { //set the begin period and end period dates $app_data['begin_period'] = $begin; $app_data['end_period'] = $end; //create the timesheet $data = $this->outputPdf($app_data); //get the current date so we can put it in the file name of the timesheet $date = date("m_d_Y"); //create the file name with the employee's name and the date $name = $app_data['name']."_".$date.".pdf"; //send it to the browser echo $this->fpdf->fpdfOutput($name, $destination = 'd'); } // if the type isn't single check to see if it is multiple and if so, generate the timesheets elseif($type == 'multiple') { //get the current date to use in the file name $date = date("m_d_Y"); //do stuff to get all apps in pdf $data = null; //create variable to hold the timesheets as we generate them //loop through the data and create a timesheet for each employee foreach ($app_data as $app) { //set up begin and end date variables for the pay period $app['begin_period'] = $begin; $app['end_period'] = $end; //generate timesheet for the current employee and store it in the data variable $data .= $this->outputPdf($app); //create a new page $data .= $this->fpdf->AddPage(); } //create the file name and include the name of the department (section) in it $name = "payroll_report_".$section."_".$date.".pdf"; //send it to the browser echo $this->fpdf->fpdfOutput($name, $destination = 'd'); }